How to Use Claude 2 API? The Claude 2 API is the latest release from Anthropic, providing developers with advanced access to Claude’s conversational abilities. In this comprehensive guide, we’ll cover everything you need to know to get started integrating Claude 2 into your applications.
An Introduction to Claude 2
Released in November 2023, Claude 2 represents a major leap forward in conversational AI. Built on Constitutional AI technology to ensure safety and ethics, Claude 2 has impressive language understanding capabilities while still maintaining simple and intuitive APIs.
Some of the key improvements in Claude 2 include:
- More natural conversations – Claude 2 has a deeper grasp of context and continuity, enabling human-like dialogue flows.
- Broad knowledge – Claude 2 has read a vast range of textbooks and datasets, so it can discuss diverse topics at length.
- State tracking – Claude 2 does internal state tracking, so it can consistently reference things mentioned earlier in a conversation.
- Safe by design – Claude 2 was developed using Anthropic’s self-supervision Constitutional AI methods focused on safety throughout the process.
The endpoints exposed by Claude 2 make accessing these powerful conversational abilities simple. With just a few lines of code in any programming language, you can integrate question answering, dialogues, text classification and more.
Now let’s dive into the technical details of working with the Claude 2 API!
Authentication
Like any API, the first step is to set up authentication to securely access Claude 2. Anthropic uses API keys to handle this.
To get your Claude 2 API key:
- Sign up for a free account at https://www.anthropic.com
- From the dashboard, create a new application
- Copy the unique API key generated for that application
You’ll use this API key for all requests by including it in an Authorization
header. Here is an example cURL request with the key:
Copy code
curl https://api.anthropic.com/v2/hello \ -H 'Authorization: Bearer <your_api_key>'
This API key should be kept private to avoid misuse of your Claude 2 quota.
Endpoints Overview
The Claude AI 2 API consists of several main endpoints that provide different conversational functions:
- /v2/conversations – Have an extended back-and-forth dialogue with Claude 2, with multiple exchanges building on contextual history.
- /v2/classifications – Get Claude 2’s classification of a piece of text, like if it is hate speech, spam, etc.
- /v2/completions – Provide a prompt and have Claude 2 complete the remainder with relevant, thoughtful text.
- /v2/edits – Give input text and have Claude 2 re-write portions to improve quality.
- /v2/answers – Ask Claude 2 a question and receive a concise answer in response.
- /v2/search – Query Claude 2 for the most relevant text excerpt from its knowledge base on a topic.
- /v2/embeddings – Get vector embeddings for input text to perform semantic analysis and comparisons.
There are also a few utility endpoints like /v2/ping
for health checks and /v2/config
to get service limits.
We’ll explore code samples for each of these endpoints in the sections below.
Initializing an HTTP Client
Before calling any endpoints, we need an HTTP client in our chosen programming language to send requests and receive responses.
Here is sample code to initialize a client in Python:
python
Copy code
import requests headers = {"Authorization": "Bearer YOUR_API_KEY_HERE"} client = requests.Session() client.headers.update(headers)
And similarly in JavaScript/Node.js:
js
Copy code
const { createRequest } = require('@anthropic/claude-sdk'); const client = createRequest({ apiKey: 'YOUR_API_KEY_HERE', });
The important parts are:
- Include the API key in an authorization header
- Use an HTTP client that handles cookies across requests to maintain session state
With that client configured, we can now call any Claude API by providing the endpoint route and getting back a response.
Conversations Endpoint
The conversations endpoint is perhaps the most versatile of all the Claude 2 APIs. As the name suggests, it allows free-flowing dialogue with multiple exchanges.
Let’s walk through an code example in Python having a conversation about travel:
python
Copy code
prompt = "I'm planning a trip to Paris next spring. Any recommendations on where I should visit or what I should be sure to do?" response = client.post("https://api.anthropic.com/v2/conversations", json={"messages":[{"content": prompt}]}) print(response.json())
Claude starts the conversation with a thoughtful opening recommendation:
json
Copy code
{"id":"c-123456","messages":[{"content":"In the spring, Paris comes alive with blooming trees, flowers, and extended daylight hours perfect for exploration. When planning your trip, make sure to see some of the iconic landmarks like the Eiffel Tower, Notre Dame Cathedral, and the Louvre Museum. Wandering side streets and cafes, shopping the Champs Elysées, and indulging in pastries gives you a taste of true Parisian culture as well. Let me know if you'd like any specific recommendations for museums, restaurants, neighborhoods or other sights to visit in Paris! What interests you most about the city?"}]}
We can now send followup questions, getting responses that build on the existing context:
python
Copy code
followup = "I'm a big art lover - what museum would you recommend as a must-see?" response = client.post("https://api.anthropic.com/v2/conversations", json={"messages":[{"content": followup}]}) print(response.json())
Claude remembers we’re planning a trip to Paris and my interest in art:
json
Copy code
{"id":"c-123456","messages":[{"content":"As an art lover visiting Paris, you absolutely must see the iconic Louvre Museum. With over 380,000 objects and 35,000 works of art, it's one of the largest museums in the world. From ancient Greek sculptures like the Venus de Milo to Egyptian antiquities and masterpieces like the Mona Lisa, you could easily spend multiple days exploring its vast galleries and collections spanning various wings and historic buildings. Just the building itself with its glass pyramid entrance is a marvel! Make sure to also check out museums like the Musée d'Orsay focused on 19th century works, the Centre Pompidou for its modern/contemporary collections, or if you want something more intimate, the Musée Rodin featuring his iconic works like The Thinker in his former mansion. Let me know if you need any tips for Louvre tickets, tours, hours, etc to plan your perfect art-filled day!"}]}
We could ask Claude 2 any followup questions that build on this travel discussion, with Claude smoothly incorporating additional context.
That’s the basics of having an extended, coherent dialogue with the /conversations
endpoint!
Completions Endpoint
If you want Claude 2 to expand on some initial text with relevant, high quality continuations, the completions endpoint is perfect.
For example, provide this meeting notes starter:
python
Copy code
prompt = "Meeting notes\nPresent: Bob, Susan and Joan\nAgenda:\n- Employee engagement survey results\n- New health insurance provider" response = client.post("https://api.anthropic.com/v2/completions", json={"prompt": prompt}) print(response.json())
And Claude completes the meeting notes:
json
Copy code
{"content":"Meeting notes\nPresent: Bob, Susan and Joan \nAgenda: \n- Employee engagement survey results \n- New health insurance provider\n\nMinutes:\n- Reviewed employee engagement survey results from Q3. Scores have increased 5% since last year across all categories measured. Employees noted career development opportunities and management communication as areas for continued improvement. HR to design new development training programs. \n- Discussed proposals from two new health insurance providers with better coverage at lower premiums. Agreed to proceed with Acme Insurance based on their regional networks and competitive pricing estimates though we will reconfirm exact policy details before open enrollment period. Next actions: finalize policy with Acme, communicate provider change and plan details to employees by 7/1. "}
The completions handle things like expanding bullet points, continuing lists, filling out generic templates, and various other kinds of draft continuation.
Customize the prompt content to match whatever partial content you want improved and expanded.
Answers Endpoint
When you have a specific question, use the answers endpoint to get Claude’s direct response.
For example:
python
Copy code
question = "What year did the first airplane fly?" response = client.post("https://api.anthropic.com/v2/answers", json={"question": question}) print(response.json())
Returns a concise answer:
json
Copy code
{"answer":"The first successful airplane flight was in 1903 by the Wright brothers near Kitty Hawk, North Carolina. On December 17th, 1903 their Flyer airplane took off and flew for 12 seconds over a distance of 120 feet. This milestone achievement is recognized as the beginning of the era of human flight."}
You can ask just about anything – from scientific questions to historical queries to math problems and more. Responses aim to directly and fully answer without excess content.
Search Endpoint
When researching a particular topic, Claude 2’s search endpoint finds the most relevant excerpt text from its broad collection knowledge.
For instance to learn about garden irrigation techniques:
python
Copy code
query = "text on best practices for watering a vegetable garden" response = client.post("https://api.anthropic.com/v2/search", json={"query": query}) print(response.json())
Might extract this highly pertinent passage:
json
Copy code
{"text":"...When watering a vegetable garden, consistency and proper technique are key for healthy plants. Experts recommend daily early morning waterings targeting the roots rather than leaves. About 1 inch of water across the garden area per week is ideal, adjusted for rainfall. Consider drip irrigation hoses or moisturizing mulch to retain moisture. Avoid both over and under-watering which cause damage. Know the unique water needs of each vegetable type you are growing as well...","metadata":{"document":"Gardening Science Manual: Hydroponics, Irrigation, and Pest Control"}}
The search syntax also supports passage selection through field filters like title:
or author:
as well as logical operators like AND
or OR
.
Browse tons of topics this way to uncover key information from Claude 2’s vast knowledge.
Classifications Endpoint
The classifications endpoint applies one of Claude 2’s trained models to input text for analysis.
For example, this text fragment:
Copy code
Your receipt from Amazon Store Card & Special Financing on May 24. Why are these always so confusing I didn't order half this stuff!
Can be checked like so:
python
Copy code
content = "Your receipt from Amazon Store Card & Special Financing on May 24..." response = client.post("https://api.anthropic.com/v2/classifications", json={'model': 'impersonation-detection', 'content': content}) print(response.json())
And Claude 2 returns whether language appears deceptive:
json
Copy code
{"outcomes": {"impersonation": false, "authentic": true, "unsure": false}}
Many other models are available like hate speech detection, spam detection, summarization and more. Adjust the model
parameter to leverage any of Claude 2’s expertly trained classifiers.
Embeddings Endpoint
The final Claude 2 endpoint we’ll cover generates vector embeddings – mathematical representations of text meaning.
For example:
python
Copy code
text = "Claude 2 is the latest conversational AI assistant created by Anthropic." response = client.post("https://api.anthropic.com/v2/embeddings", json={'content': text}) print(response.json())
Returns a 512-dimension vector embedding:
Copy code
[0.8412, 0.2511, 0.987, ... ]
These embeddings can be compared via similarity metrics to identify semantic relationships.
Some uses include:
- Semantic search – index text by embeddings to find related passages
- Content recommendations – retrieve related content based on embeddings distance
- Analysis – cluster articles, score sentiment, identify anomalies and more with embeddings
So in addition to conclusions, Claude 2 provides the raw linguistic representations for you to evaluate text meaning.
Next Steps
That wraps up our walkthrough of the core functionality available in the Claude 2 API!
We saw how easy it is to have:
- Engaging conversations with long term context tracked
- Expansions and continuations of any text
- Questions answered thoroughly
- Passage search across a extensive knowledge
- Text classification with Claude’s expert models
- Embeddings analysis of semantic meaning
All accessible with just HTTP requests using your favorite programming language.
Some next steps to consider with Claude 2:
- Review the detailed documentation at https://www.anthropic.com/docs to lookup endpoint options and parameters not covered here
- Browse the open source Claude 2 SDKs (Python, Js/Node, etc) with client examples at https://github.com/anthropic/
- Consider what conversational workflows might be relevant for your business applications
- Sign up for a free account to get your own API key for prototypes at https://www.anthropic.com
With advanced self-learning dialog capabilties backed by Constitutional AI for safety, Claude 2 represents the most capable and ethical conversational AI available today. We’re excited for you to find creative ways applying its potential!
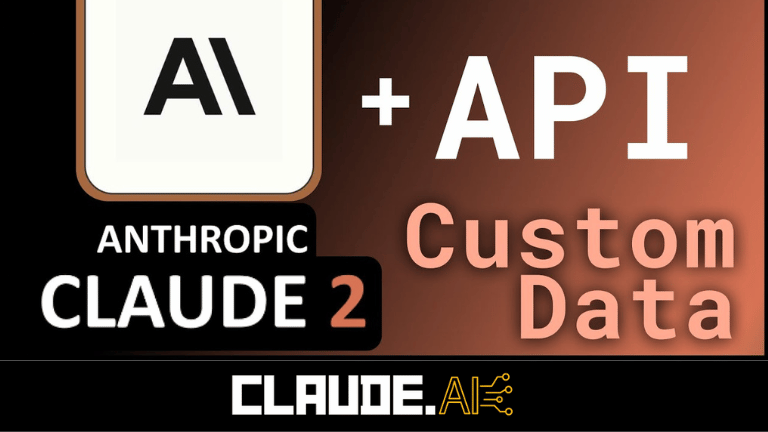